在SpringBoot中使用SpringCache
SpringCache的依赖
1 | <dependency> |
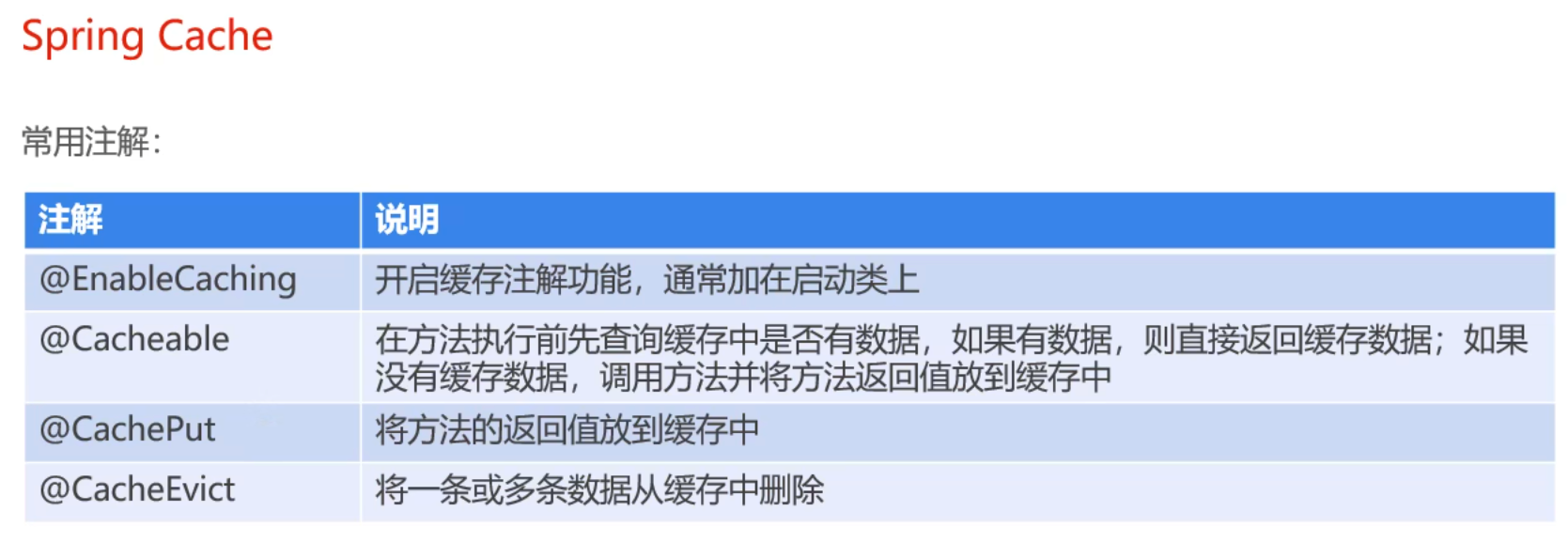

一 在启动类上加入 @EnableCaching
1 |
|
二 在需要缓存数据的方法上加入 @CachePut
1 |
|
在redis中数据的存在形式
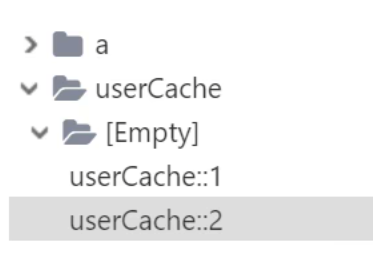
此种情况是 使用的user.id作为key, 前缀name是userCache, redis会将 :: 之间连接的关系做树状分类
三 在查询数据库前先查缓存, 在这样的方法上加入 @Cacheable, 如果缓存中没有, 则查数据库,然后自动将数据加入缓存
1 |
|
四 要清理一条或多条缓存, 则在该方法上加入 @CacheEnvict , 要清理一条则指定key = “#id”, 要清理所有cacheName下的缓存, 则加入allEntries = true
1 | // 此时指定清理一条缓存 userCache::id |